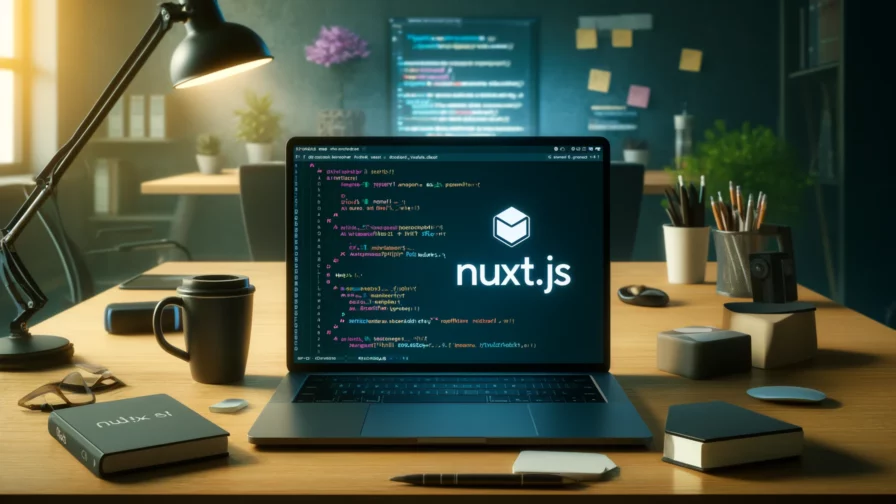
Whether you’re building a personal blog, a dynamic web application, or a complex e-commerce site, Nuxt.js offers a suite of powerful features that can streamline your development process and enhance your site’s performance.
Nuxt.js is not just another tool in the web developer’s arsenal; it’s a comprehensive framework that builds on top of Vue.js. It simplifies the development of server-side rendered (SSR) and static web applications, making it easier to create fast, scalable, and SEO-friendly websites. What sets Nuxt.js apart is its ability to manage the complexities of modern web development, allowing you to focus on writing your application logic without worrying about the underlying infrastructure.
1. Introduction to Nuxt.js
Nuxt.js is built on top of Vue.js and enhances it by providing an opinionated structure and a set of powerful features that simplify the development of complex applications. It supports server-side rendering (SSR), static site generation (SSG), and client-side rendering (CSR).
Key Features:
- Automatic Routing: File-based routing system.
- Server-Side Rendering (SSR): Improves SEO and performance.
- Static Site Generation (SSG): Generates static websites for faster load times.
- Modular Architecture: Over 50 modules for easy integration of functionalities like PWA support, authentication, and more.
- Developer Experience: Enhanced development experience with hot module replacement (HMR), detailed error reporting, and an extensive plugin system.
What is Nuxt.js mostly used for?
Real-Life Examples of Nuxt.js Usage
- E-commerce Platforms:
- Custom e-commerce sites built with Nuxt.js and RESTful Laravel backends, providing seamless shopping experiences.
- E-commerce Progressive Web Applications (PWAs) built on top of Shopify, leveraging Nuxt.js for improved performance and scalability.
- E-commerce websites leveraging Nuxt.js for dynamic content management and better SEO.
- Content and Media Websites:
- Podcast directories using Nuxt.js for server-side rendering and SEO optimization.
- Pharmacy websites integrated with WordPress API, showcasing how it can work with various content management systems.
- Corporate and Institutional Websites:
- Corporate sites for biotech companies for clean design and fast performance.
- University web portals combining Nuxt.js with Drupal-powered REST APIs for efficient data handling and presentation.
- Community and Social Platforms:
- Job boards built with Nuxt.js and Rails API, deployed to platforms like Netlify for easy management.
- Platforms connecting developers worldwide, built with Nuxt.js and Django Rest Framework, facilitating community interactions and content sharing.
- Other Notable Uses:
- Online parking management platforms use this framework for their front-end and other frameworks for the backend.
- Global pay analysis software for HR and compensation managers, showcasing Nuxt.js’s capability to handle complex data visualization and analytics.
2. Setting Up Nuxt.js
To get started with Nuxt.js, you need to have Node.js and npm (or yarn) installed.
shCopia codicenpx create-nuxt-app my-nuxt-app
cd my-nuxt-app
npm run dev
This command scaffolds a new Nuxt.js project and starts the development server.
3. Project Structure
A typical Nuxt.js project has the following structure:
arduinoCopia codicemy-nuxt-app/
├── assets/
├── components/
├── layouts/
├── pages/
├── plugins/
├── static/
├── store/
├── nuxt.config.js
- assets: Uncompiled assets such as Sass, images, or fonts.
- components: Vue components that are reusable across pages.
- layouts: Layouts for different pages.
- pages: Vue files corresponding to application routes.
- plugins: JavaScript plugins to be run before initializing the root Vue.js application.
- static: Files in this directory are served at the root of your site.
- store: Vuex store files for state management.
- nuxt.config.js: Configuration file for Nuxt.js.
4. Pages and Routing
Nuxt.js uses a file-based routing system. Any Vue file in the pages
directory automatically becomes a route.
htmlCopia codice<!-- pages/index.vue -->
<template>
<div>
<h1>Home Page</h1>
</div>
</template>
<script>
export default {
name: 'HomePage'
}
</script>
htmlCopia codice<!-- pages/about.vue -->
<template>
<div>
<h1>About Page</h1>
</div>
</template>
<script>
export default {
name: 'AboutPage'
}
</script>
These files create routes /
and /about
.
5. Layouts
Layouts provide a way to reuse common structures. Create a default layout in layouts/default.vue
.
htmlCopia codice<!-- layouts/default.vue -->
<template>
<div>
<header>
<nav>
<nuxt-link to="/">Home</nuxt-link>
<nuxt-link to="/about">About</nuxt-link>
</nav>
</header>
<nuxt />
<footer>
<p>Footer Content</p>
</footer>
</div>
</template>
This layout is automatically applied to all pages unless a different layout is specified.
6. Server-Side Rendering (SSR)
Nuxt.js handles SSR out of the box. To fetch data on the server side, use the asyncData
method in your page components.
htmlCopia codice<!-- pages/index.vue -->
<template>
<div>
<h1>{{ title }}</h1>
</div>
</template>
<script>
export default {
async asyncData({ $axios }) {
const response = await $axios.$get('https://api.example.com/data')
return { title: response.title }
}
}
</script>
7. Static Site Generation (SSG)
To generate a static site, add the following to nuxt.config.js
:
javascriptCopia codiceexport default {
target: 'static',
}
Then run:
shCopia codicenpm run generate
This command generates a static version of your site in the dist
directory.
8. Plugins
Nuxt.js plugins allow you to run custom code before initializing the root Vue.js application.
javascriptCopia codice// plugins/axios.js
export default function({ $axios }) {
$axios.onRequest(config => {
console.log('Making request to ' + config.url)
})
}
// nuxt.config.js
export default {
plugins: ['~/plugins/axios.js']
}
9. Vuex Store
Nuxt.js supports Vuex for state management. Create a store by adding files to the store
directory.
javascriptCopia codice// store/index.js
export const state = () => ({
counter: 0
})
export const mutations = {
increment(state) {
state.counter++
}
}
Use the store in components:
htmlCopia codice<template>
<div>
<button @click="increment">Increment</button>
<p>{{ counter }}</p>
</div>
</template>
<script>
export default {
computed: {
counter() {
return this.$store.state.counter
}
},
methods: {
increment() {
this.$store.commit('increment')
}
}
}
</script>
10. Middleware
Nuxt.js supports middleware functions that run before rendering a page or layout.
javascriptCopia codice// middleware/auth.js
export default function({ store, redirect }) {
if (!store.state.authenticated) {
return redirect('/login')
}
}
// nuxt.config.js
export default {
router: {
middleware: 'auth'
}
}
11. Modules
Nuxt.js has a rich ecosystem of modules to extend its functionality. For example, to add Google Analytics:
shCopia codicenpm install @nuxtjs/google-analytics
javascriptCopia codice// nuxt.config.js
export default {
modules: [
'@nuxtjs/google-analytics'
],
googleAnalytics: {
id: 'UA-XXXXXXX-X'
}
}
12. Deployment
Nuxt.js applications can be deployed to various platforms such as Vercel, Netlify, or traditional servers. For a static site:
shCopia codicenpm run generate
Upload the contents of the dist
directory to your preferred hosting provider.
Conclusion
Nuxt.js is a powerful framework that simplifies the development of Vue.js applications by providing a structured environment with built-in support for server-side rendering, static site generation, and many other features.
Its modular architecture and extensive ecosystem make it an excellent choice for building modern web applications.
Here’s a breakdown of the main benefits of Nuxt.js:
- Robust and Flexible: Ideal for various projects, from personal blogs to complex e-commerce sites.
- Built-in Features: Streamlines development with SSR and SSG capabilities enhancing performance and SEO.
- Modular Architecture: Allows easy extension of functionality.
- Intuitive Structure: Simplifies maintenance and scalability.
- Community Support: Strong community and comprehensive documentation.
- Focus on Quality: Enables developers to concentrate on creating high-quality applications without infrastructure concerns.