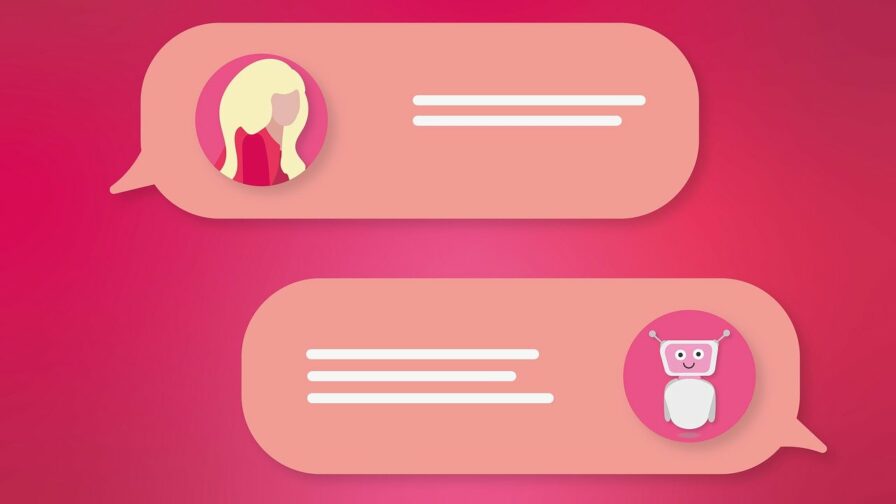
Building a ChatBot with Python is easier than you may initially think. Chatbots are extremely popular right now, as they bring many benefits to companies in terms of user experience.
In this guide, we’re going to look at how you can build your very own chatbot in Python, step-by-step.
Before we get to that, though, let’s learn a little bit about chatbots and how they work.
What is a Chatbot?
A chatbot is a piece of AI-driven software designed to communicate with humans. Chatbots can be either auditory or textual, meaning they can communicate via speech or text.
Chatbots can be used in a variety of ways. In many cases, they’ll be used to supplement human agents in sales or back, where they can perform tasks such as scheduling appointments, helping customers to perform self-service, or capturing the details of leads.
They have other uses though. For example, ChatGPT for Google Sheets can be used to automate processes and streamline workflows to save data input teams time and resources.
Over 30% of people primarily view chatbots as a way to have a question answered, with other popular uses including paying a bill, resolving a complaint, or purchasing an item.
There are two main types of chatbot:
Rule-based chatbots
Rule-based chatbots interact with users via a set of predetermined responses, which are triggered upon the detection of specific keywords and phrases. Rule-based chatbots don’t learn from their interactions, and may struggle when posed with complex questions.
AI-based chatbots
AI-based chatbots learn from their interactions using artificial intelligence. This means that they improve over time, becoming able to understand a wider variety of queries, and provide more relevant responses. AI-based chatbots are more adaptive than rule-based chatbots, and so can be deployed in more complex situations.
What is Python?
There’s no doubt: today, Python is one of the most widely used computer programming languages.
Many developers use Python and its different frameworks and libraries to build websites and software, but it can also be used to conduct data analysis, automate tasks, and create a Python data pipeline.
Rank | Change | Language | Share | 1-year trend |
---|---|---|---|---|
1 | Python | 27.43 % | -0.2 % | |
2 | Java | 16.19 % | -1.0 % | |
3 | JavaScript | 9.4 % | -0.1 % | |
4 | C# | 6.77 % | -0.3 % | |
5 | C/C++ | 6.44 % | +0.2 % |
ChatterBot
ChatterBot is a Python library designed to respond to user inputs with automated responses. It uses various machine learning (ML) algorithms to generate a variety of responses, allowing developers to build chatbots that can deliver appropriate responses in a variety of scenarios.
Because it’s built using ML algorithms, the chatbot will also be able to improve its performance as it learns over time. Chatterbot also features language independence, meaning that it can be used to train chatbots in multiple programming languages.
A chatbot built using ChatterBot works by saving the inputs and responses it deals with, using this data to generate relevant automated responses when it receives a new input. By comparing the new input to historic data, the chatbot can select a response that is linked to the closest possible known input.
Before starting, it’s important to consider the storage and scalability of your chatbot’s data. Using cloud storage solutions can provide flexibility and ensure that your chatbot can handle increasing amounts of data as it learns and interacts with users. It’s also essential to plan for future growth and anticipate the storage requirements of your chatbot’s conversations and training data. By leveraging cloud storage, you can easily scale your chatbot’s data storage and ensure reliable access to the information it needs.
Moreover, the more interactions the chatbot engages in over time, the more historic data it has to work from, and the more accurate its responses will be.
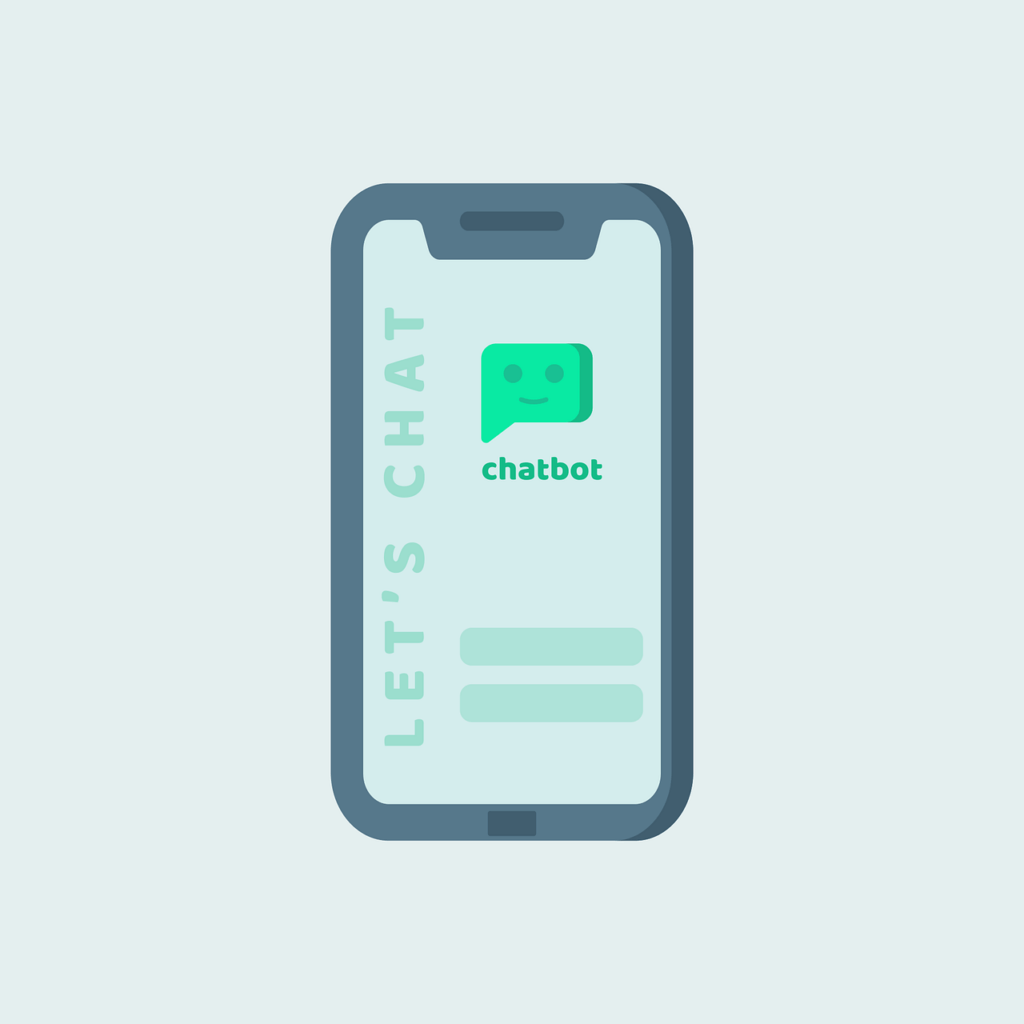
Prerequisites
We’ve tried to make this guide as easy to follow as possible, so you should be able to build your own chatbot in Python, regardless of whether you’re a pro developer with a working knowledge of software such as open source data modelling tools, or a complete novice.
However, there are a few key concepts you should be clued up on before you begin, including:
- General Python functions
- Substring checks
- Substring replacements
- File input/output
- Lists and tuples
Before starting, you should import the necessary data packages and initialize the variables you wish to use in your chatbot project. It’s also important to perform data preprocessing on any text data you’ll be using to design the ML model.
Creating the testing and training datasets before you begin will also help you save time later on.
We’ll be using the ChatterBot library to create our Python chatbot, so ensure you have access to a version of Python that works with your chosen version of ChatterBot.
If you need a quick refresher on Python and its use before you begin, there are plenty of online resources that will show you how to learn Python for data engineering, or a multitude of other purposes.
Recommended read: Document Understanding With Large Language Models on Google Cloud
Developing Your Own Chatbot From Scratch
Now that we’re armed with some background knowledge, it’s time to build our own chatbot.
- Prepare dependencies
The first step is to install the ChatterBot library in your system. It’s recommended that you use a new Python virtual environment in order to do this.
To achieve this, write and execute this command in your Python terminal:
pip install chatterbot
pip install chatterbot_corpus
If you wish to upgrade to ChatterBot’s latest development version, execute the command:
Pip install - -upgrade chatterbot_corpus
Pip install - -upgrade chatterbot
Once these steps are complete your setup will be ready, and we can start to create the Python chatbot.
- Import classes
The next step is to import classes.
Classes are code templates used for creating objects, and we’re going to use them to build our chatbot.
There are two classes we’ll need to download to do this: ChatBot from chatterbot, and ListTrainer from chatterbot.trainers.
To download these, execute these commands:
from chatterbot import ChatBot
from chatterbot.trainers import ListTrainer
Code language: JavaScript (javascript)
- Train the chatbot
The chatbot you’re building will be an instance belonging to the class ‘ChatBot’.
Create a new ChatterBot instance, and then you can begin training the chatbot.
Training the chatbot will help to improve its performance, giving it the ability to respond with a wider range of more relevant phrases.
You can begin by executing the following command:
my_bot = ChatBot(name=’Chatty’, read_only=True,
logic_adapters=
[‘chatterbot.logic.MathematicalEvaluation’,’chatterbot.logic.BestMatch’])
Code language: PHP (php)
Let’s break down that command so we understand how we’re training the chatbot.
‘name=’ refers to the name of your chatbot. We’ve called this one Chatty, but feel free to choose something more original!
If you’re planning to set up a website to give your chatbot a home, don’t forget to make sure your desired domain is available with a check domain service.
For example, if you’re developing an AI-driven chatbot for an ecommerce website, you can train it to provide product recommendations, answer customer inquiries about orders and shipping, and assist with the checkout process.
The command ‘read_only=True’ means that the chatbot’s ability to learn after this training has been disabled. This is optional, depending on the purpose of the chatbot.
The command ‘logic_adapters’ provides the list of resources that will be used to train the chatbot.
This chatbot is going to solve mathematical problems, so ‘chatterbot.logic.MathematicalEvaluation’ is included. This logic adapter checks statements for mathematical equations. If one is present, a response is returned containing the result.
The logic adapter ‘chatterbot.logic.BestMatch’ is used so that that chatbot is able to select a response based on the best known match to any given statement.
In order for this to work, you’ll need to provide your chatbot with a list of responses.
Here are just a few of the strings you can train your chatbot to learn:
animals = [“aardvark”, “badger”, “cat”, “dog”, “elephant”]
For clarity, you can also place each string on a separate line:
small_talk = [“hi!”
“how\’re you?”
“what\’s up?”
“glad to hear that”
“i\’m chatty the chatbot. Do you have a maths question?”]
Code language: PHP (php)
We’ll need to train it to be able to recognise and solve maths problems if it’s going to be asked them, for example:
math_talk_1 = [‘pythagorean theorem’,
‘a squared plus b squared equals c squared.’]
Your chatbot is now ready to engage in basic communication, and solve some maths problems.
- Communicate with the chatbot
Now you can start to play around with your chatbot, communicating with it in order to see how it responds to various queries.
It’s important to remember that, at this stage, your chatbot’s training is still relatively limited, so its responses may be somewhat lacklustre.
You should take note of any particular queries that your chatbot struggles with, so that you know which areas to prioritise when it comes to training your chatbot further.
- Train the chatbot further
Now that you’ve got an idea about which areas of conversation your chatbot needs improving in, you can train it further using an existing corpus of data.
A corpus is a collection of authentic text or audio that has been organised into datasets. There are numerous sources of data that can be used to create a corpus, including novels, newspapers, television shows, radio broadcasts, and even tweets.
ChatterBot provides some corpora that can be used for this purpose:
from chatterbot.trainers import ChatterBotCorpusTrainer
corpus_trainer = ChatterBotCorpusTrainer(my_bot)
corpus_trainer.train(‘chatterbot.corpus.english’)
Code language: JavaScript (javascript)
ChatterBot offers corpora in a variety of different languages, meaning that you’ll have easy access to training materials, regardless of the purpose or intended location of your chatbot.
If you wish, you can even export a chat from a messaging platform such as WhatsApp to train your chatbot. Not only does this mean that you can train your chatbot on curated topics, but you have access to prime examples of natural language for your chatbot to learn from.
Once your chatbot is trained to your satisfaction, it should be ready to start chatting.
Don’t forget to test your chatbot further if you want to be assured of its functionality, (consider using software test automation to speed the process up).
Congratulations, You’ve Built a Chatbot in Python
The method we’ve outlined here is just one way that you can create a chatbot in Python. There are various other methods you can use, so why not experiment a little and find an approach that suits you.
The chatbot we’ve built is relatively simple, but there are much more complex things you can try when building your own chatbot in Python. If it sparks your interest, then learn how deep learning works. You can build a chatbot that can provide answers to your customers’ queries, take payments, recommend products, or even direct incoming calls.
Keep working at it, and see what you can achieve.