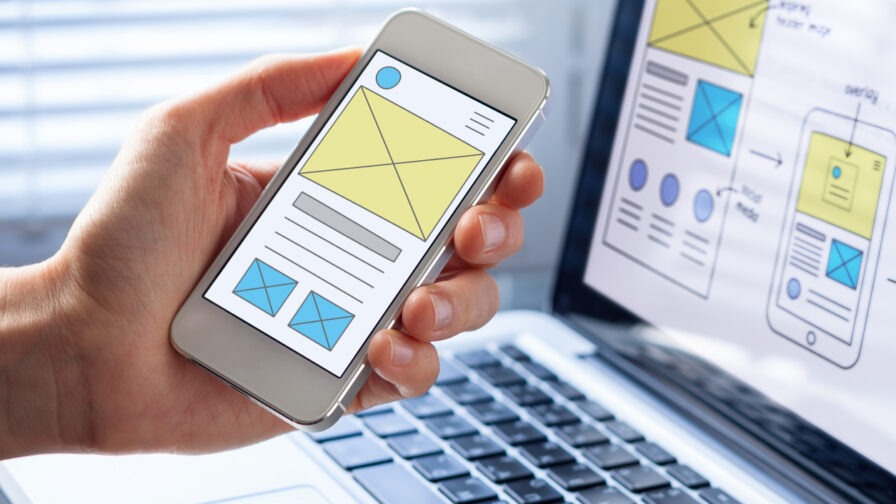
Angular is not dead, despite the constant calls and warnings in that direction, it is more alive than ever, especially with its last version. And creating a Single Page Application, with multiple routes helps you with the performance and load time of your page.
In this simple tutorial, we will explain when it is convenient to use SPA and how to do it easily with Angular.
What is a Single Page Application, and what’s used for?
Single plage applications are great for creating engaging and unique user experiences for website visitors. These sites or web applications dynamically rewrite an existing web page with new information. These websites don’t load new pages entirely and don’t refresh the pages. Instead, the code is retrieved by the browser using a single page load. Only a part of the web page will be changed with every click of the mouse.
Where can we see SPA?
Lots of businesses are using SPAs to offer better user experiences. Some popular single plage applications include:
- PayPal
- Netflix
- Gmail
- Google Maps
- AirBnB
Advantages and Disadvantages
The main advantage of single page applications is that they have a high load speed. Unlike MPAs or multi-page applications, SPAs don’t secure all their pages to their core. That means they take less time and effort to maintain security. This helps to quicken the load speed of the websites. It is also worth noting that SPAs download everything upfront, and that means the back-and-forth is eliminated. The new information will always be downloaded as a single page. It is also faster to navigate single page application sites since they have higher caching capabilities. Caching works better with SPAs as these sites request data from the server just one time.
Another advantage of single page applications is that they are able to work offline. When the internet connection is restored, the local data will be synced with the server of the website. These websites also offer cross-platform functionality. They can work on different operating systems on different browsers.
SPAs also offer a great user experience. You won’t have to click through links to access different pages and will simply need to scroll through the pages. SPAs are especially good for mobile users.
Although single page applications are efficient, they have some disadvantages. First, they can hurt your SEO since they use JavaScript. Search engines like Bing and Yahoo work better with HTML format. Another issue with SPAs is that they make it hard to track website metrics. This is because Google Analytics will not be able to determine which pages or content are more popular among readers.
Single page applications also offer production deployment advantages. They are very easy to deploy in production and to version. The application is only one index.html file and has a CSS bundle and a JavaScript bundle. You can upload these three static files to a content server like Amazon S3, Apache, Nginx, or Firebase Hosting. The backend server can be built with different technology. Node, Java, and PHP are some popular choices.
Prerequisites in AngularJS
To build a single page application in AngularJS, you will need a basic understanding of the following:
- TypeScript
- HTML
- CSS
- Angular CLI
You also need to install Node and Node Package Manager.
Step 1: Create a Module
The first step is to create a module. This is a container for the different components of the application. Some of these components are the controller and service. You can create a module by issuing the following command:
Var app = angular.module( ‘myApp’ , []);
Code language: PHP (php)
Since Angular uses MVC architecture, every app is made up of different modules.
Step 2: Define a simple controller
Next, you have to define a simple controller. Do this with the following code:
app.controller('FirstController', function($scope) {
$scope.message = 'Hello from FirstController';
});
Code language: PHP (php)
Step 3: Include AngularJS in the Code
At this point, you have to use the module and controller in the HTML code. You have to first add the Angular script and app.js that you have developed. It is also necessary to specify the module in the ng-app tribute. In the ng-controller tribute, you must specify the controller.
You can ensure that everything is configured properly on localhost. Also, check whether AngularJS is operational.
Step 4: Use $routeProvider service from ngRoute module
AngularJS ngRoute module provides routing, directives and deep linking services for angular applications. For this step, you have to download the angular-route.js script which has the ngRoute module. It is also possible to use the CND in your application to include the routing file.
After bunding the file into your application, you can add the ngRoute module in the Angular JS application. This should be added as a dependent module as you can see below:
angular.module(‘appName’, [‘ngRoute’]);
The ngView directive can be used to show HTML templates or views in the indicated routes. Whenever the route is altered, the view will change as per the configuration of the $route service.
To configure the routes, you have to use $route provider. You can configure it using the ngRoute config(). The config() will have a function and uses the $routeprovider as the parameter. The routing configuration will be in the function. $routeprovider can either use the when() or otherwise() method. With the when() method, you will use a path and route as the parameters. The path is simply the part of the URL that is seen after the hash sign.
For example, in the URL randompage.com/index.html#/home, the path is ‘/home’.
The route has two properties, and these are the templateURL and controller. TemplateURL will define the HTML template that AngularJS should load and display in the div with the ngView directive. On the other hand, the controller will define the controllers that will be used in the HTML template. Once you load the application, the path will be matched with the part of the URL after the hash sign.
In case the route paths don’t match the provided URL, the browser will use the path specified in the otherwise() function. Using the same example of randompage.com/index.html#/students, you can set the path to ‘/students’ and the otherwise() function to ‘/home’. If the ‘/students’ path doesn’t match anything, it will go into otherwise() condition, and the page will be redirected to home.html.
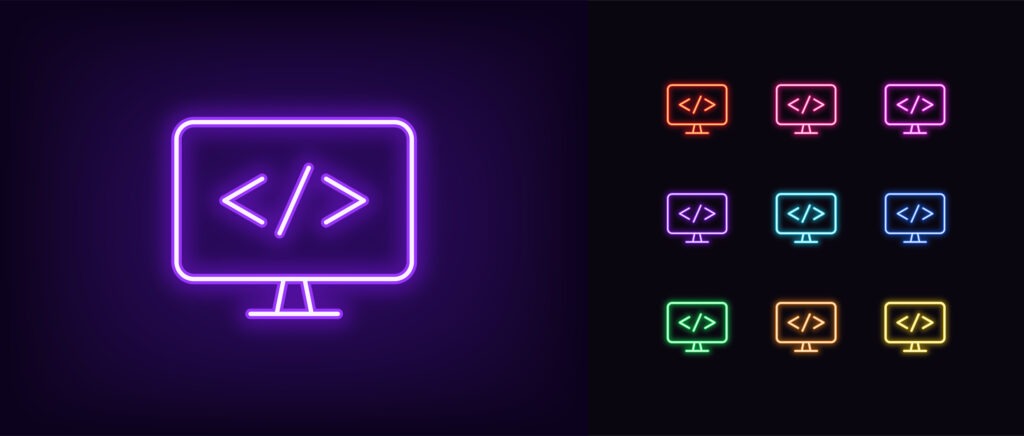
Step 5: Configure the pages that will be active
Finally, you have to configure the pages that will be active. You should save all the files in the same directory and open index.html on your browser. After generating an HTML layout for your site, you can use the ngView directive to determine where the HTML for each page will be shown in the layout. Configure the pages with proper HTML format. For example, for the about page, you should use H1 for the title ‘About’. You should also add links to the HTML to help with navigation to the configured pages.
Conclusions
Single page applications offer many advantages. They load faster and offer a great user experience. They are also easy to deploy in production and to version. These applications only load a single HTML page, and only part of the page gets updated with every click of the mouse. This is different from multi-page applications as they load a new page with every click. Lots of popular modern applications use the concept of SPAs. They include Gmail, Facebook, Twitter, and Netflix. You can build SPAs with Angular.