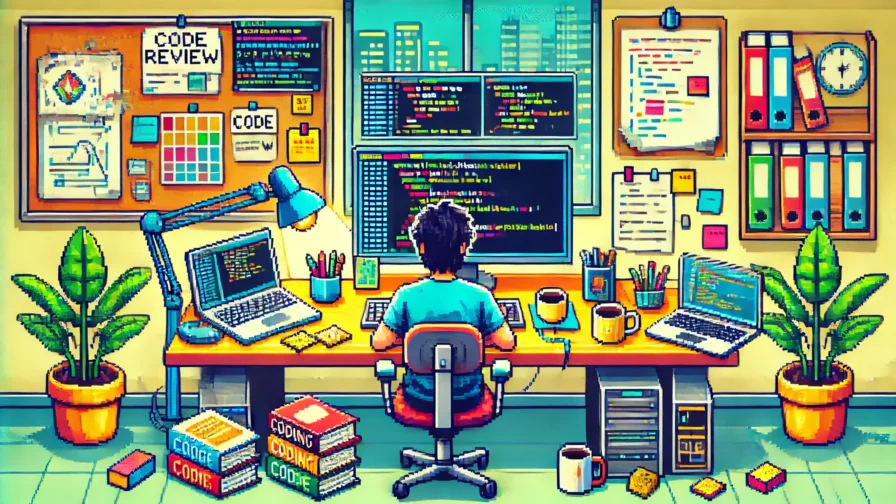
Code reviews are essential for maintaining high-quality software. They play a critical role in saving company resources, enhancing code performance, ensuring scalability, and preventing future bugs. Effective reviews provide opportunities for team members to catch errors early, improve the overall quality of the code, and share knowledge. However, if not done correctly, they can quickly become a source of frustration.
This article explores the benefits of code reviews in terms of cost savings, code quality, performance, and scalability. It also highlights common mistakes to avoid for a more effective review process, and shares some of the most popular review tools today.
Why Reviews Matter
- Quality Assurance: reviews help identify bugs and issues early in the development process. According to SmartBear, reviews can catch 70-90% of defects in the reviewed code when conducted effectively, significantly reducing the number of defects that reach production.
- Knowledge Sharing: By involving multiple developers in the review process, companies ensure that knowledge about code structure and implementation details is distributed across the team. This is crucial for onboarding new developers and maintaining consistency across the codebase.
- Standards Compliance: Reviews enforce coding standards and best practices, making the codebase more maintainable and readable in the long term.
- Security: Early detection of potential security vulnerabilities is another key benefit of code reviews. By identifying and addressing these issues early, teams can mitigate the risk of security breaches later in the development cycle.
How Companies Review Their Code
- Pull Requests: In many companies, developers submit their code changes through pull requests, which are then reviewed by peers or senior developers before being merged into the main codebase. Tools like GitHub and GitLab are widely used to facilitate this process.
- Automated Tools: Automated code review tools, such as Codebeat and Snyk, help identify common issues and potential vulnerabilities, allowing human reviewers to focus on more complex problems.
- Pair Programming: Some teams adopt pair programming, where two developers work together at one workstation, enabling continuous, real-time code review and collaboration.
- Review Checklists: Checklists are often used to ensure that all critical aspects of the code are reviewed. These might include checks for readability, functionality, performance, and security.
Data Supporting Code Reviews
- Defect Density Reduction: Studies show that code reviews can significantly reduce defect density. For instance, a SmartBear study indicated that reviewing 200-400 lines of code at a time can yield a defect detection rate of up to 90%.
- Cost Savings: Hewlett Packard’s historical data revealed that incorporating reviews saved more money than resolving defects post-release, highlighting the economic benefits of early defect detection.
- Maintenance Efficiency: Research suggests that code reviews can cut maintenance costs by up to 50%, underscoring the long-term benefits of maintaining a high-quality codebase from the outset.
These practices and tools ensure that code reviews are not only a mechanism for improving code quality but also a means to enhance team collaboration and maintain high development standards.
Main Mistakes in Code Reviews
1. Skipping Tests
Mistake: Skipping tests during reviews is a frequent issue. Reviewing tests can be tedious, leading some developers to bypass them.
Solution: Always give tests the same level of attention as the rest of the code. Tests are critical for catching bugs early and ensuring the code behaves as expected.
Example (Python):
def add(a, b):
return a + b
def test_add():
assert add(2, 3) == 5
assert add(-1, 1) == 0
assert add(0, 0) == 0
Code language: JavaScript (javascript)
Review these tests to ensure they cover all edge cases and possible scenarios.
Common Question: Why are tests so important in a code review?
Answer: Tests verify that the code works as intended. They help identify bugs early, which reduces the cost and effort of fixing issues later in the development cycle. Skipping tests can lead to missed bugs and unreliable software.
2. Reviewing Only Newly Added Code
Mistake: Focusing solely on new or changed code can lead to a fragmented understanding of the codebase.
Solution: Review the entire codebase context to ensure that new changes integrate seamlessly with existing code.
Example (JavaScript):
// New Function
function multiply(a, b) {
return a * b;
}
// Existing Function
function add(a, b) {
return a + b;
}
Code language: JavaScript (javascript)
Review both functions to ensure consistency in error handling, naming conventions, and overall logic.
Common Question: How can I ensure a thorough review without spending too much time?
Answer: Use a checklist to ensure you cover all important aspects, such as functionality, readability, maintainability, and performance. Tools like linters and static analysis can help automate some checks, saving time for more in-depth review aspects.
3. Rushing the Review
Mistake: Conducting a rushed review can result in missed issues and lower code quality.
Solution: Allocate sufficient time for thorough reviews and avoid last-minute checks.
Example (Java):
// Original Code
public int divide(int a, int b) {
return a / b;
}
// Reviewed Code with error handling
public int divide(int a, int b) {
if (b == 0) {
throw new IllegalArgumentException("Divider cannot be zero");
}
return a / b;
}
Code language: PHP (php)
Take time to ensure proper error handling and consider all edge cases.
Common Question: What is a good time allocation for a code review?
Answer: It depends on the complexity of the code, but a good rule of thumb is to spend at least one hour per 400 lines of code. Ensure that the review is done in a distraction-free environment to maintain focus.
4. Ignoring Design and Architecture
Mistake: Overlooking the overall design and architecture in favor of focusing on minor syntax issues.
Solution: Evaluate the impact of changes on the overall system design and architecture.
Example (C#):
// Ensure that the new service fits into the existing architecture.
public class NewService : BaseService {
// Implementation
}
// Check for consistency with existing services and design patterns.
Consider how new code impacts scalability, maintainability, and adherence to design patterns.
Common Question: Why should design and architecture be a focus during reviews?
Answer: Proper design and architecture ensure that the codebase remains scalable, maintainable, and efficient. Ignoring these aspects can lead to technical debt and make future changes more difficult and costly.
5. Providing Unclear Comments
Mistake: Leaving vague or ambiguous comments can frustrate the developer and lead to miscommunication.
Solution: Offer clear, specific, and actionable feedback.
Example (Ruby):
# Vague comment
# "Fix this"
def calculate_total(price, tax)
price + tax
end
# Clear comment
# "Ensure tax is always a positive number to avoid incorrect totals"
def calculate_total(price, tax)
raise ArgumentError, "Tax must be positive" if tax < 0
price + tax
end
Code language: PHP (php)
Provide detailed comments that explain the reasoning behind the feedback.
Common Question: How can I provide constructive feedback without discouraging the developer?
Answer: Focus on the code, not the coder. Use positive language and explain why changes are necessary. Offer suggestions rather than just pointing out problems, and acknowledge good practices and improvements.
Human, Automated, or Hybrid Code Review?
Code reviews can be conducted in various ways, each with its own set of advantages and disadvantages. Here are the main approaches:
1. Manual (Human) Reviews
Description: In this approach, developers manually review each other’s code. This method is highly detailed and can catch complex logic errors.
Advantages:
- Detailed and nuanced feedback
- Encourages knowledge sharing and team collaboration
Disadvantages:
- Time-consuming
- Can be inconsistent depending on the reviewer’s expertise and mood
2. Automated Reviews
Description: Automated code review tools (like SonarQube, Codacy, or ESLint) analyze code for style violations, potential bugs, and other issues.
Advantages:
- Consistent and fast
- Can catch common mistakes and enforce coding standards
Disadvantages:
- Limited to predefined rules and patterns
- May miss context-specific issues
3. Hybrid Reviews
Description: Combines manual and automated reviews to leverage the strengths of both methods. Automated tools handle routine checks, while human reviewers focus on logic, design, and architecture.
Advantages:
- Efficient and comprehensive
- Balances speed and detail
Disadvantages:
- Requires integration of tools and processes
- Can still be time-consuming
The Role of AI in Code Reviews
AI-powered tools like GitHub Copilot are changing the landscape of code reviews by assisting in code completion, suggesting improvements, and even generating code snippets.
AI-Powered Reviews
Description: AI tools assist developers by providing code suggestions, catching common mistakes, and generating code snippets based on the context of the project.
Advantages:
- Increases productivity by reducing repetitive tasks
- Provides instant feedback and suggestions
Disadvantages:
- May not understand the full context or complex logic
- Requires oversight to ensure accuracy and relevance
Common Questions about AI in Code Reviews
Q: How reliable are AI tools like GitHub Copilot in code reviews?
A: AI tools are quite reliable for generating code snippets, catching common mistakes, and providing suggestions. However, they are not a substitute for human judgment and should be used to augment, not replace, manual reviews.
Q: Will AI tools replace human code reviewers?
A: AI tools are unlikely to replace human reviewers entirely. They are best used to assist with repetitive tasks and provide suggestions, while human reviewers handle the nuanced aspects of code quality, design, and architecture.
Q: How can AI tools improve the code review process?
A: AI tools can speed up the review process by automating routine checks, providing instant feedback, and suggesting code improvements. This allows human reviewers to focus on more complex and high-level issues, leading to a more efficient and thorough review process.
Popular Tools and Assets for Code Reviewing
- GitHub – Popular Git repository hosting with integrated review features.
- JetBrains Space – Comprehensive team collaboration tool with built-in code review.
- Bitbucket – Git solution with native Jira integration for enhanced collaboration.
- Rhodecode – Centralized code management for Git, Mercurial, and SVN.
- GitLab – Complete DevSecOps platform with robust code review and reporting.
- Codebeat – Open-source automated code review tool for various programming languages.
- Azure DevOps – Microsoft’s CI/CD, agile, and DevOps toolset with Azure Repos.
- Collaborator – SmartBear’s tool for comprehensive code and document review.
- Snyk – Developer security platform focusing on dependency management.
- Veracode – Security-centric tool providing static analysis and penetration testing.
Conclusion
Avoiding common mistakes in code reviews and choosing the right approach can significantly improve the effectiveness of the process. Reviewing code is not just a technical necessity but also a valuable opportunity for learning and collaboration among team members.