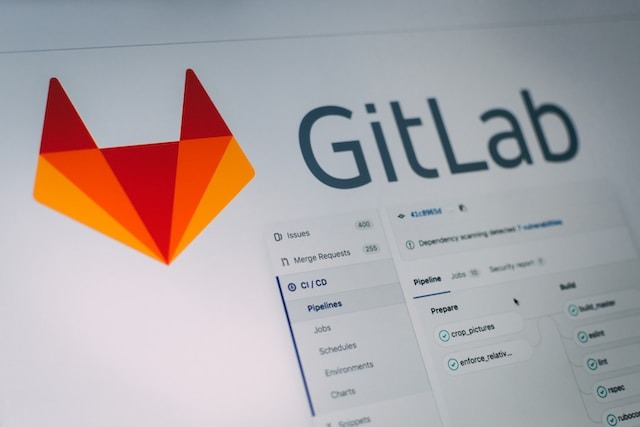
As developers, every line of code we write counts towards forming the backbone of our applications. Yet, being human, any line of code we write could contain bugs that cause problems for our customers and our teams. This is where code reviews come in. Code review checklists serve as a safety net against these unintentional missteps.
But code reviews aren’t just about identifying errors – they enhance the code’s readability, maintainability, and overall performance. With a well-structured checklist in hand, you can make your code reviews more effective and thorough.
After all, one of the hallmarks of great code is readability. A fellow developer should be able to dive into your codebase and understand it without requiring a tour guide.
This article offers an in-depth look into code reviews, outlining their significance and providing you with a comprehensive checklist to improve your developer work.
Code Review Tool | Description |
---|---|
GitHub | A popular web-based platform for hosting and reviewing code. It offers built-in code review features, such as pull requests and code comments. |
GitLab | An integrated platform for the entire DevOps lifecycle, including code review features like merge requests and inline code comments. |
Bitbucket | A Git repository management solution that includes code review capabilities through pull requests and code comments. |
Phabricator | An open-source suite of web-based tools for code review, bug tracking, and other software development tasks. |
Gerrit | A web-based code review system for Git repositories, often used for projects with strict quality control requirements. |
Crucible | A code review tool by Atlassian, designed to work with various version control systems, such as Git and Mercurial. |
Review Board | An open-source web-based code review tool that can be integrated with multiple version control systems. |
Upsource | A code review and repository browsing tool by JetBrains, designed to work seamlessly with their IntelliJ IDE. |
Collaborator | A peer code review tool that supports multiple version control systems and integrates with IDEs like Visual Studio and Eclipse. |
Reviewable | A web-based code review platform that integrates with GitHub, providing an enhanced code review experience. |
GitKraken | A Git client that includes built-in code review features and integrates with popular Git hosting platforms. |
What is a code review?
A code review is when another developer examines and critiques your code. The goal is to identify possible errors, improve code quality, and ensure you’re following best practices. Beyond pinpointing mistakes, the reviewer is also making sure that the agreed-upon requirements have been met.
Most development teams use git or GitHub, where a developer submits their proposed changes in a pull request (PR). The developer has a version of the code they’ve amended, and they’re requesting that the team “pull” those changes into the main version. One or more team members review the PR to ensure it meets quality standards. They might leave comments with questions or requested changes before eventually signing off on the PR.
Image sourced from jetbrains.com
A code review could involve multiple reviewers depending on how important it is that this code is flawless. Reviews are a great opportunity for knowledge sharing, mentorship, and collaboratively refining the codebase. Team members exchange feedback and share insights to boost the overall code quality.
Why should you always review code?
A code review isn’t just about checking boxes. Successive reviews make the code better over time. As well as inspecting new changes, a code review gives developers a chance to look at existing code with fresh eyes and see if they’re up-to-date with current best practices.
If you’re building omnichannel contact center software, for example, there are a lot of components that cover different channels. If they’re not regularly updated, this can cause problems where some files are written in a completely different style to what the team is used to.
Here’s a breakdown of why you should use a code review checklist:
- Early detection: The first step towards refining software is pinpointing areas that are either problematic or have room for improvement. With an all-inclusive checklist, reviewers are better suited to spot small mistakes before they balloon into more pressing challenges.
Image sourced from akfpartners.com
- Strengthen team collaboration: Code reviews provide a collaborative space between senior and junior team members, facilitating mentorship opportunities for newer developers. Senior team members can impart valuable insights, while the newer members bring a fresh pair of eyes to existing code and practices.
- Boost productivity: In software development, time is valuable. A code review checklist spots problems early, saving time that would otherwise be used to fix them later. This avoids high-pressure situations where teams might think about sacrificing code quality to meet deadlines. Simply put, it’s a way to code more efficiently, saving time and effort.
- Promote best practices: Codifying best practices in a checklist provides immediate access to a tried-and-tested knowledge base, ensuring you stick to coding standards and maintain an organized code base. It reminds you to work towards consistent coding norms, follow design templates, and elevate performance.
A review code checklist is invaluable to both seasoned developers who’ve navigated similar projects, and newcomers who may still be learning the best practices for their specific language.
How to prepare for a code review
Before diving into the code review checklist, follow these steps to ensure an effective review process. Let’s take a look:
- Implementation: Start by gaining a clear understanding of the feature that’s being implemented. What problem does it claim to solve? What does the developer think their code is doing? This foundational understanding will provide context to the code changes and make the review more meaningful.
- Verify compilation and testing: Before diving deeper into the intricacies of the code, ensure that the basics are in place. The code should compile without any errors. Additionally, it should pass unit tests that ensure each individual function is performing as expected. Any code that fails these fundamental checks might not be ready for a detailed review.
- Refer to the related task details: Every pull request is usually associated with a ticket or task that outlines what the problem is, and what might need to be done to solve it. There might be extra considerations in the ticket or conversation linked to it that aren’t obvious. Familiarise yourself with this ticket. This will give you insights into the requirements, expectations, and constraints that the developer might have encountered.
By following these preliminary steps, you set the stage for a thorough, efficient, and constructive code review checklist.
The ultimate 10-point code review checklist
When reviewing a colleague’s work, we assess the code’s design, style, functionality, and testing. Given its many stages, reviewers need to adopt a systematic and detailed approach. This 10-point code review checklist will help you get it right every time.
1. Functional consistency
Every pull request has a mission: solving a specific issue, or adding a particular feature. The first question to ask is whether the PR meets the agreed-upon requirements for fulfilling its purpose.
To assess functionality, consider the following questions:
- Have any requirements not been met?
- How well have the requirements been met?
- Can you think of any edge cases that this code isn’t prepared to deal with?
- Are there any additional features that users or other developers might appreciate?
2. Code readability and structure
A piece of code can be functional, but as you hire backend developers it’s going to cause problems down the road if it’s not readable. One of the telltale signs of problematic “legacy code” is that nobody on the current team knows how to read it. Clear coding conventions act as signposts, helping developers navigate new code now and helping future developers navigate old code in years to come.
Structured indentation and consistent naming conventions ensure the code is organized, easy to skim, and intuitive to understand. Adding comments throughout, especially in complex pieces of code, gives colleagues the context they need to understand what code is supposed to be doing.
3. Performance implications
When reviewing code for performance, it’s best to look for areas that could hamper your system’s efficiency. Adopt QA testing best practices to ensure software quality. Here are some key points to consider:
- Bottlenecks: Check for any sections of the code that might be slowing down the overall execution. Many languages have built-in affordances for timing the execution of specific functions in testing. Such areas could severely affect the user experience, especially during peak usage.
- Unnecessary repetitions: Look for loops or recurring processes that could be streamlined or optimized. Removing redundant steps can significantly boost performance.
- Resource-intensive operations: Identify areas that consume excessive resources, such as memory or processing power. There may be lighter alternatives or optimizations possible for these operations.
The ultimate goal is to ensure that the code uses resources judiciously. With optimal performance, you improve your system’s responsiveness and safeguard user experience.
4. Error handling and logging
When examining the code, prioritize robust error handling. This means actively scanning for mechanisms that are designed to catch and address errors. A well-structured system should be capable of managing unexpected issues without causing disruptions.
Additionally, the code should have logging features in place to facilitate debugging and troubleshooting. It’s equally important to ensure that error messages are clear and descriptive, and offer actionable insights for those addressing the issues. Proper error handling and detailed logging are pivotal for smooth system operations and resolution.
5. Security concerns
Integrating security into every phase of the development process is vital – it’s why DevSecOps is important in cybersecurity. Make sure that your process includes audits and testing around security, and that your best practices include specific points on common security issues: protections against denial of service attacks, SQL injections, or people accessing parts of the system they shouldn’t be able to.
Key things to consider include:
- Vulnerable patterns: Are there any coding patterns that might make the application susceptible to threats?
- Data leaks: Are there any potential data leaks that could expose sensitive information?
- Insecure data handling: Is the data being managed securely and appropriately?
- Other security compromises: Are there code sections that might jeopardize the system’s security in any way?
The goal is to safeguard your application and user data from any looming threats or malicious attacks. By prioritizing security, you bolster your application’s integrity and uphold the trust of users.
6. Testing and coverage
When reviewing code, consider the depth and breadth of the associated tests. Are there tests that validate the functionality of new features? And if new code impacts existing features, have the current tests been modified to reflect these changes?
As you delve into the testing landscape of your code, consider including the following:
- Test cases: New features or modifications should ideally be accompanied by tests that confirm its functionality. These are usually unit tests that check specific functions.
- Impact on existing tests: When new code influences existing features, current tests must be updated or modified to mirror these changes.
- Coverage: It’s crucial to gauge the extent of the testing. Questions to consider include: Are the primary functionalities rigorously tested? Do the tests consider potential edge cases?
- Integration tests: It’s not just about unit tests. Check if integration tests have been performed to check that the new code plays nicely with the rest of the codebase.
When you’re working on big B2B products like call center systems technology, thorough integration testing is essential. These are complex codebases, and users stand to lose business if something goes wrong.
As well as including integration testing in their process, teams will often release new code gradually to catch any errors that the review missed. Testing the app is a holistic process that goes beyond the code review, often taking 20% of developer time.
7. Code duplication
When going through your checklist, keep an eye out for repetitive blocks of code. Often, newer team members might not be aware of existing functions or libraries within the codebase. As a result, they could introduce redundant functions.
Redundancy can make the codebase harder to maintain and introduce inconsistencies or bugs when changes are made. As a reviewer, find opportunities to reduce the number of lines of code (LoC). This will make the software more consistent and efficient, as well as making it more readable for other team members.
8. Code clarity and dependencies
Examine the code to ensure optimal use of current libraries, frameworks, or components, and verify that dependencies are handled aptly and kept updated. Your checklist could include the following prompts:
- Does the code effectively use established libraries, frameworks, or components?
- Are those dependencies up-to-date?
- Have any redundant or out-of-date dependencies been handled appropriately?
- Are the dependencies reliable, under active maintenance, and meet quality standards?
Priority #1 at this stage is compatibility The aim is to ensure that the new additions or modifications work seamlessly with what’s already in place.
9. Documentation and comments
Make sure the code is well-documented. This includes block comments with high-level overviews, inline comments addressing specific lines of code, and comments that explain what each function does.
- Are there comments that clarify challenging or non-intuitive portions of the code?
- Are there comprehensive comments or docstrings attached to functions, methods, and classes?
- Does the codebase include overarching documentation for sophisticated modules or components?
- Has the documentation been consistently revised and kept current?
Beyond just serving as a reference, the primary aim of both documentation and comments is to foster clarity. By ensuring these elements are in place and well-curated, future developers are afforded a smoother experience.
10. Compliance with coding standards
Compliance with coding standards is vital in preserving the integrity and quality of a codebase. These norms, whether set by an organization or tailor-made for a project, create a unified framework for you to follow.
It’s essential to maintain these standards consistently. Such dedication not only streamlines code reviews but also minimizes error potential, ensuring the overall robustness of your codebase.
Wrapping up
Code reviews play a central role in software development, acting as both a quality checkpoint and a platform for continuous learning. Make sure your reviews are thorough and consistent with a code review checklist. As developers, let’s embrace these guidelines and strive for excellence in every line of code we make.