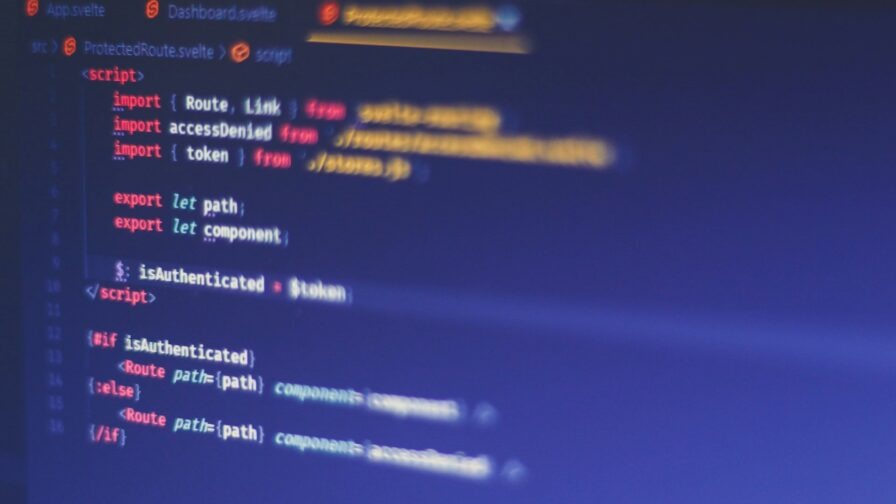
Looking for a JavaScript guide? Discover everything about the leading language in web development: it’s flexible, fun, and extremely powerful. But did you know that it has hidden features and “secret” capabilities that not everyone is aware of? In this article, I will take you on a 360-degree journey, exploring the various aspects of the language, analyzing the answers to the most common questions about JavaScript. Not only will we analyze the code and web development, but we will also explore its applications in other areas, to provide you with a complete overview of this programming tool.
JavaScript Guide: The Basics
What is JavaScript?
JavaScript is a widely used programming language for the development of interactive web applications. It is an interpreted scripting language that runs client-side, i.e., in the user’s web browser. JavaScript was first introduced in 1995 and has since become one of the essential tools for creating dynamic and engaging user experiences on the Internet.
What is JavaScript used for?
JavaScript is a versatile language that offers a wide range of functionality for web development. It is primarily used to add interactivity to web pages, allowing developers to create elements such as drop-down menus, dynamic input forms, slideshows, animations, and much more. JavaScript is also used to manage asynchronous requests to the server, allowing content on a page to be updated without having to reload it completely. Additionally, complex web applications can be created using JavaScript frameworks and libraries like React, Angular, and Vue.js.
Is JavaScript a front-end technology?
Yes, JavaScript is mainly used as a front-end programming language for web development. This means that it runs in the user’s browser and allows direct interaction with the elements of the web page, dynamically modifying them and responding to user events. However, JavaScript can also be used as a back-end programming language using a runtime environment like Node.js. In this way, JavaScript can be used to create complete web applications, managing both the client-side and server-side logic of the application.
How long does it take to learn JavaScript?
The time it takes to learn JavaScript can vary from person to person, depending on prior programming experience and commitment to study. However, in general, learning the basics of JavaScript can take several weeks to several months of consistent practice. It is essential to start with the fundamental concepts of the language, such as syntax, variables, operators, and flow control, and then progress to more advanced topics like functions, objects, arrays, and the use of libraries and frameworks.
What can JavaScript do?
JavaScript offers a wide range of features and possibilities for web development. Some of the things you can do with JavaScript include manipulating the DOM (Document Object Model) to dynamically update elements on a web page, handling events to respond to user actions, interacting with external APIs to fetch and send data, creating animations and smooth transitions, validating forms, implementing custom business logic, and much more. Additionally, with the help of libraries and frameworks like React, Angular, or Vue.js, JavaScript can be used to create complex and modern web applications.
JavaScript Frameworks and Libraries
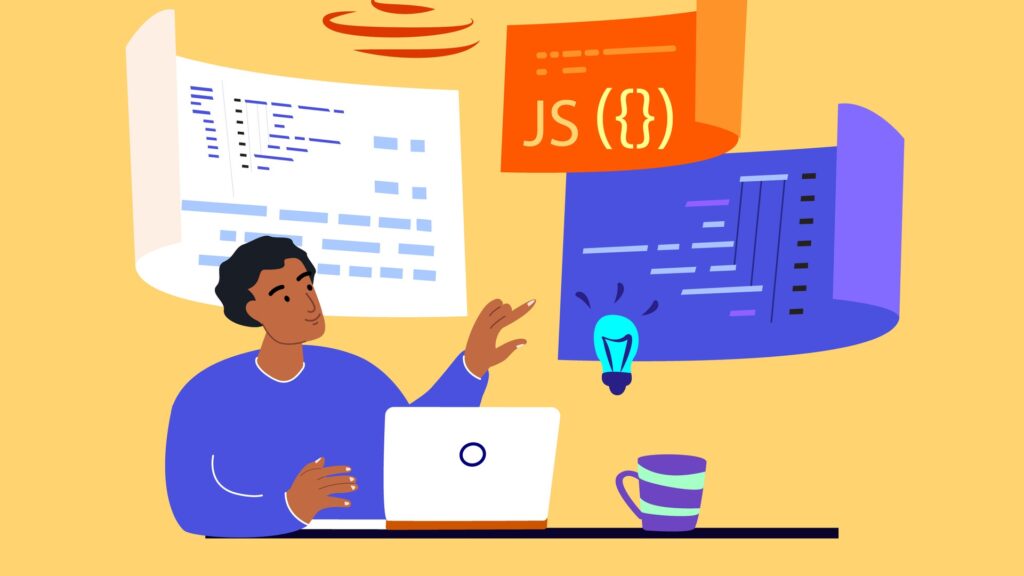
Are Java and JavaScript the same?
Despite the similar names, Java and JavaScript are two distinct programming languages. Java is a high-level programming language often used for server-side application development, Android mobile applications, and other complex software. On the other hand, JavaScript is primarily a scripting language used for web development. The syntax, language structure, and use cases are significantly different between the two. Java also requires explicit compilation before being able to run the code, while JavaScript is an interpreted language executed directly in the browser.
Differences between React and JavaScript?
React is an open-source JavaScript library widely used for creating interactive user interfaces. Therefore, the main difference between React and JavaScript is that React is a library that relies on the JavaScript language to provide specific tools and conventions for developing dynamic user interfaces. React uses a component-based structure to allow developers to create modular and reusable interfaces. While JavaScript is a general-purpose programming language, React is a specialized library for user interface development.
What’s the difference between TypeScript and JavaScript?
TypeScript is a superset of JavaScript that adds the ability to use static types during the development phase. Unlike JavaScript, which is an interpreted programming language, TypeScript requires a compilation phase to convert the code into valid JavaScript. The addition of static types allows for detecting errors during the development phase and improving code readability and maintainability. JavaScript, on the other hand, is more flexible and can be written more concisely, but does not offer the same type checking tools provided by TypeScript.
What’s the difference between Node.js and JavaScript?
JavaScript is the programming language used in both front-end and back-end web development. However, Node.js is a runtime environment that allows you to run JavaScript on the server side. Node.js provides a set of features that enable developers to create scalable and high-performance server-side applications. This includes the ability to handle HTTP requests, access the filesystem, communicate with databases, and more. While JavaScript runs in the browser, Node.js extends JavaScript’s capabilities to the server, opening the door for full-stack development using a single language.
Are ECMAScript and JavaScript the same?
JavaScript is a programming language based on ECMAScript standards. ECMAScript is a standard that defines the syntax and behavior of the JavaScript language. JavaScript is the practical implementation of ECMAScript and also includes additional features provided by browsers and other runtime environments. So, in simple terms, JavaScript is the common name for the programming language, while ECMAScript is the standard that defines the technical specifications of the language. This means that JavaScript versions correspond to different ECMAScript versions, such as ECMAScript 5, ECMAScript 6 (or ES2015), and so on.
JavaScript Guide: Possibilities, Getting Started, and Enabling
How to enable JavaScript?
JavaScript is enabled by default in most modern web browsers. However, in some circumstances, it might be disabled by the user for security reasons or other personal preferences. To enable JavaScript, the user can follow these general directions:
Chrome: In the address bar, type “chrome://settings/content/javascript” and make sure the option “Allow all sites to run JavaScript” is enabled.
Firefox: In the address bar, type “about:config“, search for “javascript.enabled” and ensure the value is set to “true“.
Edge: Click on the three dots in the top right corner, select “Settings“, then select “Website” in the left column and ensure the “JavaScript” option is enabled.
How to know if JavaScript is enabled on Chrome, Firefox, and Edge?
To check if JavaScript is enabled in your browser, you can follow these steps:
Chrome: Open a new tab, type “chrome://settings/content/javascript” in the address bar, and make sure the option “Allow all sites to run JavaScript” is enabled.
Firefox: Open a new tab, type “about:config” in the address bar, search for “javascript.enabled” and verify that the value is set to “true“.
Edge: Open a new tab, click on the three dots in the top right corner, select “Settings“, then select “Website” in the left column and ensure the “JavaScript” option is enabled.
How to include JavaScript in a web page?
To include JavaScript in a web page, you can use the HTML <script>
element. There are several ways to do this. Internal script: You can include the JavaScript code directly in the HTML using the src attribute or by writing the code between the <script></script>
tags. For example:
<script>
// Inline JavaScript code
alert("Welcome!");
</script>
Code language: HTML, XML (xml)
External script: You can include an external JavaScript file using the src attribute. For example:
<script src="script.js"></script>
Code language: HTML, XML (xml)
Make sure to place the <script>
element within the <head>
section or before the closing </body>
tag, so that the browser loads and executes the code correctly.
Write a JavaScript code snippet Here is an example of a JavaScript code snippet that shows how to calculate the sum of two numbers and display it in the browser’s console:
// Variable declaration
var number1 = 5;
var number2 = 10;
// Calculating the sum
var sum = number1 + number2;
// Displaying the sum in the console
console.log("The sum is: " + sum);
Code language: JavaScript (javascript)
This example shows a simple use of variables, operators, and output in the console using JavaScript. You can customize this snippet and experiment with other JavaScript concepts to create more complex and functional code.
Can JavaScript only work within the web browser?
No, JavaScript is not limited to just the web browser. In addition to being executed within the browser as a client-side scripting language, JavaScript can also be used server-side through the use of runtimes like Node.js. This allows developers to use JavaScript to create complete web applications, including server-side operations such as database access, data processing, and request handling. JavaScript has become a very versatile and ubiquitous language in the software development ecosystem.
Is security important when programming in JavaScript?
Yes, security management is crucial when programming in JavaScript. Since JavaScript runs in the user’s browser, it is subject to potential threats such as malicious script injection (XSS) and unauthorized access to user data. To ensure secure programming in JavaScript, it is important to adopt the following best practices:
- Validating input data to prevent XSS attacks.
- Using protective methods such as escaping special characters and sanitizing data.
- Implementing authentication and authorization mechanisms to control access to sensitive data.
- Keeping JavaScript libraries and frameworks updated to fix any security vulnerabilities.
- Using secure HTTPS connections to protect communications between the browser and the server.
Security management is a fundamental part of developing safe and reliable JavaScript applications, and it is essential to consider these aspects throughout the entire development process.
Which editors to choose to write JavaScript code?
There are several text editors and integrated development environments (IDEs) that are widely used for writing JavaScript code. Some popular editors among developers include:
Visual Studio Code: A free and highly customizable code editor that provides JavaScript support through advanced features such as syntax highlighting, autocompletion, debugging, and integration with development tools.
Sublime Text: A lightweight yet powerful text editor with a wide range of plugins and customizations available for JavaScript development.
Atom: An open-source, highly customizable code editor that offers a variety of packages and themes for editing and developing JavaScript.
WebStorm: A commercial IDE that provides a wide range of JavaScript-specific features such as code completion, debugging, refactoring, and more.
The choice of editor often depends on the developer’s personal preferences and the features required for the project. It’s essential to find an editor that suits your needs and provides a smooth and efficient development experience.
If you’re enjoying this JavaScript guide, be sure to check these other articles!
Why use Typescript for your next project
A Quick Guide to the NextJS Framework
Does JavaScript implement both object-oriented and functional paradigms?
Yes, JavaScript is a programming language that implements both the object-oriented and functional paradigms. As a flexible and dynamic language, JavaScript allows developers to write code using both paradigms depending on the needs of the project.
Object-oriented paradigm: JavaScript supports the creation of objects, classes, and inheritance of methods and properties through prototyping. You can define classes and create new objects based on these classes using the class keyword. Additionally, JavaScript provides a wide range of methods to manipulate objects, such as Object.create(), Object.assign(), and many others.
Functional paradigm: JavaScript allows the use of functions as first-class citizens, which means they can be passed as arguments, assigned to variables, and returned by other functions. It also supports concepts like using pure functions, data immutability, and declarative programming.
The combination of object-oriented and functional paradigms in JavaScript enables developers to write more flexible and modular code, adapting to the different needs of the application.
What are the most important recent innovations in JavaScript?
In recent years, JavaScript has continued to evolve, and numerous innovations and improvements have been introduced. Some of the most important ones are:
Arrow Functions: Arrow functions are a concise syntax for defining functions in JavaScript. They are characterized by the notation () => {} and offer a more compact way to declare anonymous functions.
Promises and async/await: These features have been introduced to simplify the handling of asynchronous operations in JavaScript. Promises make it easy to manage the execution flow of asynchronous operations, while async/await provides a more readable and concise syntax for writing asynchronous code.
ES6 Modules: The introduction of modules in ECMAScript 6 (ES6) has simplified the organization and reuse of JavaScript code. Modules allow you to import and export functions, variables, and classes between different JavaScript files.
Classes: ECMAScript 6 introduced a clearer and more convenient syntax for defining classes in JavaScript, making the object-oriented approach more similar to that of other programming languages.
Template literals: Template literals are a new syntax for creating strings with dynamic values. They allow you to embed JavaScript expressions within strings delimited by backticks (`).
These are just a few of the most significant innovations introduced. The language continues to evolve and add new features to improve developer productivity and enable the creation of more powerful and flexible applications.
We hope this JavaScript guide was useful in clearing your doubts and getting started with this amazing language for web development!!