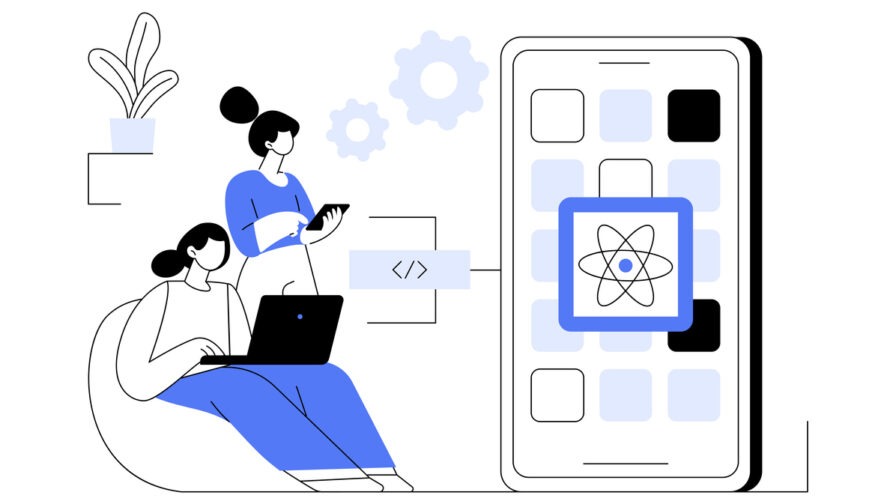
Welcome to the world of React! If you are new to web development or want to expand your knowledge, this article is specifically designed for you. We will begin with an overview of what the React library is, its goals, and its distinctive strategy compared to traditional JavaScript. We will also explore how to install, launch, and create a React component. Moreover, we will examine in detail all the key terms of the React world, which will provide you with a solid foundation for working with React more effectively. Finally, we will discuss the importance of Redux in React applications and find out what it means to use React Native for mobile app development. So, without further ado, let’s dive into the world of React!
What is React?
React is a JavaScript library designed to facilitate the creation of interactive and scalable user interfaces (UI). It was developed by Facebook and is used by many large companies, such as Instagram and Airbnb, to build complex and high-performance web applications. Thanks to its component-based architecture and declarative approach, React promotes code reusability, simplifying the management and maintenance of complex UIs. Moreover, the extensive developer community offers ongoing support, providing resources, documentation, and tools to ease the learning process and development with React.
Who created the React Library?
React was created by a team of Facebook developers led by Jordan Walke. Walke’s main goal was to create a tool that simplified the development of complex user interfaces, reducing complexity and improving performance. His work was published in 2013, and since then, React has gained popularity in the developer community.
How long does it take to learn React?
The time it takes to learn React depends on various factors, such as your programming experience level, your familiarity with JavaScript, and the time you can dedicate to studying and practicing. However, with the necessary commitment and dedication, you can acquire a solid basic knowledge of React in a relatively short period. To begin, having a solid understanding of JavaScript is helpful, as React is written and based on this programming language.
If you are already familiar with the fundamental concepts of JavaScript, it will be easier for you to tackle React. Otherwise, you may want to spend some time improving your JavaScript skills before fully diving into React. An essential aspect of learning React is practice. Creating small projects or implementing code examples will help solidify your knowledge and familiarize yourself with key React concepts, such as components, state, props, and the component lifecycle. Remember that learning React is an ongoing process, as the library is constantly updated, and new features are introduced over time. It is advisable to stay up-to-date with the latest React versions and continue exploring new resources and projects to improve your skills.
React’s Strategy and Advantages Over Traditional JavaScript
React’s development strategy is based on a fundamental concept called “Virtual DOM” (Document Object Model). The traditional Document Object Model (DOM) is a hierarchically structured representation of an HTML or XML document that allows the browser to interact with the elements present on the web page. The DOM considers the document as a tree of objects, where each element, attribute, and text is represented as a node within the tree.
Unlike traditional JavaScript, which directly manipulates the native components of the DOM (HTML elements and nodes, CSS styles, texts) whenever there are changes, React creates a virtual representation of them. This representation is compared with the actual version of the DOM and applies only the necessary changes. This approach allows React to optimize update operations and improve the overall performance of the application, as well as offering a more fluid and modern development experience.
The main advantages of React include:
Efficiency: Thanks to the use of the Virtual DOM, React can make updating the user interface more efficient and faster compared to traditional JavaScript. Changes are applied only where necessary, minimizing the computational load.
Code Reusability: React promotes strong modularity through its component-based approach. Components can be reused in different parts of the application, thus reducing code duplication and simplifying maintenance.
Active Community: React has a large and active community of developers, which means you can find many resources, documentation, and online support. This makes it easier for new developers to learn React and get help when needed.
Scalability: React is designed to create scalable applications. Its modular architecture and efficient management of user interface updates make it easy to handle large-scale projects without sacrificing performance.
Recommended video: Maya Shavin – Are we React-ing wrongly?
How to Install React Library
To start developing with React, the first thing to do is to install the correct development environment. Here are the steps to follow to install React:Prerequisites: Make sure you have Node.js installed on your computer. You can download it for free from the official Node.js website and follow the appropriate installation instructions for your operating system.
Project Initialization: Open the terminal and navigate to the directory where you want to create your React project. Once inside the directory, run the following command to initialize a new React project using Create React App:
npx create-react-app project-name
This command will create a new folder with the name of your project and install all the necessary dependencies to start developing with React.
Starting the Development Server: Once you have successfully completed the previous step, navigate to your React project folder using the command:
cd project-name
Once inside the project folder, run the following command to start the React development server:
npm start
This will launch the development server and automatically open your React project in your default browser. Now you are ready to start developing your React application!
How to Create a React Component
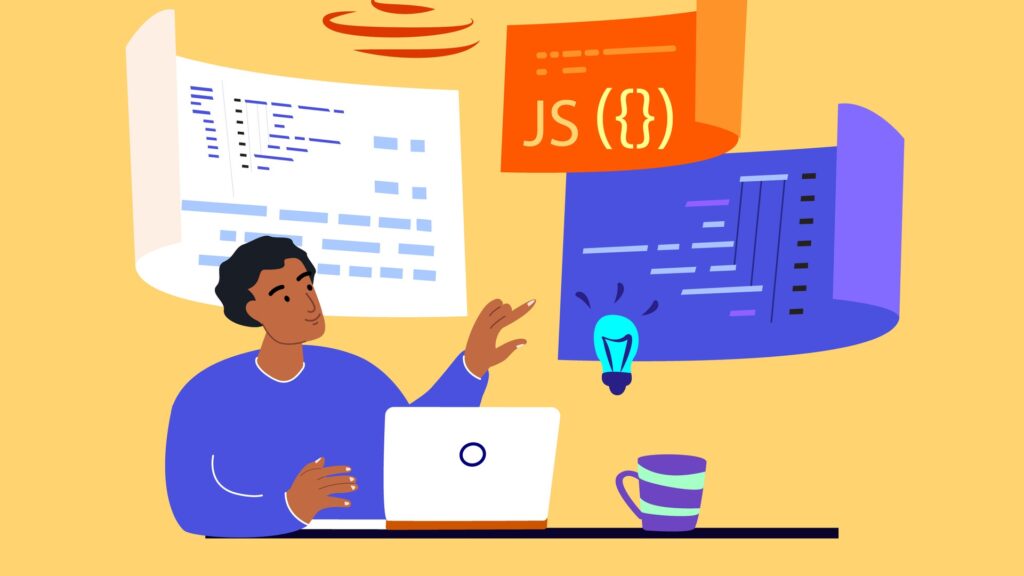
Creating components is a fundamental aspect of development with React. Follow these steps to create your first React component: In your React project folder, find the “src” directory and create a new folder inside called “components”. This will be the location where you store your components. Inside the “components” folder, create a new file with the “.js” extension and give your component a meaningful name. For example, you could call it “MyComponent.js”. Open the “MyComponent.js” file with your preferred code editor and start defining your React component. Here’s an example of what it might look like:
import React from 'react';
const MyComponent= () => {
return (
<div>
<h1>Hello, I am your React component!</h1>
<p>This is my first creation with a React Component.</p>
</div>
);
};
export default MyComponent;
Code language: JavaScript (javascript)
Once you have defined your component, you can use it within other components or in the main application. For example, you can add your component to the “App.js” file so that it is displayed in your application.
At the beginning of the file, import the component you created in step 3. Be sure to specify the correct file path if it is located in a subdirectory. For example, if your component’s file is in the components folder, the import will be:
import MyComponent from './components/MyComponent';
Code language: JavaScript (javascript)
Now you can use the MyComponent component within the App function and render it. Replace the existing code inside the App function with the following:
function App() {
return (
<div className="App">
<h1>My React application </h1>
<MioComponente />
</div>
);
}
export default App;
Code language: JavaScript (javascript)
Now, when you view your application in the browser, you will see your MyComponent component rendered inside the div element with the App class.
Remember that you can use the components created in App.js or in any other component within your React application. You can compose the user interface by combining different components and defining the desired structure.
React Library: Key Concepts
During development with React library, you will encounter some fundamental key terms that are important to understand. These terms define the core concepts of React and will help you create more effective applications. Let’s look at them in detail:
- Components: Components are the building blocks of React. They can be thought of as modular bricks that make up the user interface. Components represent self-contained parts of the application and can be reused flexibly. They are written as JavaScript functions or classes and can have state and properties.
- State: State represents the data managed internally by a component. It is one of the key aspects that make React powerful. The component can access and modify its own state, and when the state changes, React automatically handles updating the user interface to reflect those changes.
- Props: Props, short for “properties,” are mechanisms used to pass data from a parent component to a child component. Props are immutable and allow you to configure the behavior and appearance of components.
- Component Lifecycle: Components in React have a lifecycle that includes various key moments, such as mounting, updating, and unmounting. During these stages, you can perform specific operations, such as initializing data or cleaning up resources. Understanding the component lifecycle is essential for effectively managing operations.
- JSX: JSX is a JavaScript extension syntax that allows you to define the user interface structure in a declarative way. With JSX, you can write HTML-like code within React components, making the creation of the user interface more intuitive and readable.
- Events: Events in React allow you to handle user interactions, such as clicking a button or entering text in an input field. You can listen for events using specific attributes within React components and define actions to be performed when the event occurs.
- Conditional Rendering: Conditional rendering refers to the ability to render different elements or components based on specific conditions. You can use control structures, such as if statements or ternary operators, to determine what to display in the user interface based on certain conditions.
Understanding these fundamental key terms will give you a solid foundation for working with React more effectively. As you gain experience in development with React, you can fully leverage the potential of these features to create dynamic and interactive applications.
React Ecosystem: Redux and React Native
The React ecosystem is quite comprehensive, providing you with many tools to enhance your applications and improve the development experience. Among these tools, Redux and React Native stand out.
What is Redux, and why is it important in React applications?
Redux is a state management library often used alongside React for developing complex applications. While React takes care of managing the user interface, Redux focuses on managing the global state of the application. The need for Redux becomes evident when the React application becomes more complex and requires shared state across multiple components. By using Redux, you can centralize the application’s state in a single global “store,” which can be accessed and modified by any component within the application. The main advantages of using Redux include:
- Centralization of state: Redux allows for a centralized application state, simplifying state management and synchronization across components.
- Ease of debugging: With Redux, you can log all actions performed in the application, making debugging and tracking state changes much easier.
- Application scalability: Using Redux, you can easily manage complex applications with large amounts of data and components, thanks to its action and reducer-based architecture.
- Reversible actions: Redux supports undoable and redoable actions, allowing for simple undo and redo operations within the application.
What is React Native?
React Native is a development framework that enables you to create mobile apps for iOS and Android using JavaScript and React. By using React Native, developers can share code between different platforms, thus reducing the time and effort needed to develop and maintain separate native apps for iOS and Android. The main features and benefits of React Native include:
- Cross-platform development: With React Native, you can write a single codebase in JavaScript and share it across iOS and Android, reducing development time and the need to maintain two separate codebases.
- Native performance: React Native uses native components to render the user interface, providing performance similar to a native app. This allows developers to create fast and responsive apps.
- Code reuse: Much of the code written for a React Native app can be reused for other apps, saving time and effort in developing new applications.
- Extensive developer community: React Native has a large and active developer community, providing support, resources, and ready-to-use components, simplifying the development process. With React Native, developers can create native mobile apps while maintaining the flexibility and power of React and JavaScript. It is a popular choice for mobile app development, especially when rapid time-to-market and code sharing across platforms are required.
Conclusion: We Love React Library!
In this article, we explored React, a JavaScript library that has revolutionized how we develop user interfaces. We discussed React’s fundamental concepts, the origin of the framework, and its development strategy. We also highlighted the advantages React offers compared to traditional JavaScript, such as efficiency, code reusability, an active community, and scalability. We hope this introduction to React has inspired you to dive deeper into this powerful development tool. Happy coding!