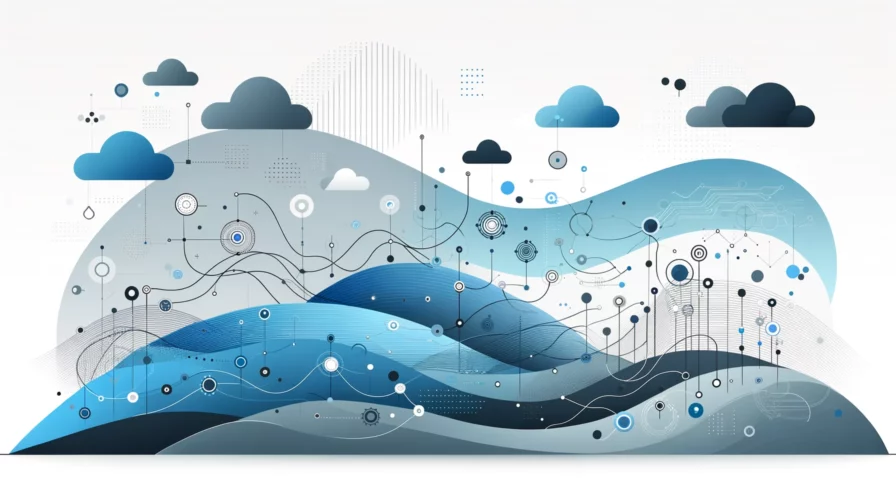
Introduction
In today’s tech-heavy world, blending different technologies to work seamlessly together is crucial. That’s where Cross-Domain Interoperability (CDI) steals the spotlight—it’s essential for any forward-thinking business and a crucial skill for system engineers. As companies pile on more computing environments and applications, ensuring smooth interaction becomes non-negotiable.
This guide takes a deep dive into CDI, laying out some straightforward yet powerful solutions. We’ll show you the ropes with some core strategies and approaches that can help you get your systems in sync.
What is Cross Domain Interoperability and Why It’s Key
Cross-domain interoperability refers to the ability of different systems, platforms, or domains to work together within a larger network, sharing data and processes without recurring modifications. The main capability of CDI is to synchronize incoming information with multiple listeners. This is crucial, as it enables organizations to leverage new and legacy technologies cohesively. The benefits of implementing CDI are profound, ranging from enhanced decision-making based on comprehensive data insights to improved operational efficiency and flexibility in adopting new technologies.
Diving Deeper Into Cross-Domain Interoperability
Mismatched data formats, clashing protocols, different architectural standards…
When different systems work together, they don’t all process information at the same speed as they all have different response SLAs, even if it’s the same data. This difference in processing times is a key part of what makes it complex to get these systems to work smoothly together.
Navigating the path to solid Cross-Domain Interoperability (CDI) can be tricky with hurdles, but getting a grip on some key interoperability principles such as loose coupling, service orientation, and agreeing on protocols—can really smooth things out.
It’s important to note that CDI goes beyond mere patterns and conventions; it begins with a detailed analysis of the diverse systems that need to communicate. After understanding these systems, the most suitable technologies are selected—sometimes an ApiGateway suffices, and other times an Event Driven data orchestrator is necessary. This practical approach links CDIF’s theoretical foundations to real-world applications, making the framework more relatable and easier to understand.
Cross-Domain Interoperability Patterns, Tools and Approaches
Before diving into CDI patterns and tools, it’s interesting to explore the pattern standardization attempt by the Cross-Domain Interoperability Working Group (CDIF) and the various aspects of their FAIR approach, as it provides useful insights and context.
Patterns are your main ally when it comes to implementing and streamlining processes and ensuring smooth data flow across systems. Let’s take a look at some of the main examples.
Patterns:
Event Driven Design: This design pattern promotes the production, detection, consumption, and reaction to events. EDD also boosts scalability thanks to asynchronous event-driven communication for the different systems involved. Systems can also be decoupled and react to real-time data changes dynamically.
When to use Event-Driven Design
As a typical didactic example, let’s consider an e-commerce platform where customers place orders for products. With event-driven design, when a customer successfully completes a purchase (the event), it triggers the generation of an “order placed” event by the producer. This event is then consumed by various downstream services such as inventory management, payment processing, and shipping. Each of these services reacts accordingly, updating inventory, processing payment, and initiating the shipping process.
Here’s a pseudo-code example with a producer and a consumer interacting using a simple event-driven model in Python. The snippet shows how to set up an event class and subscribe a handler to the event. The Event class is central to managing and dispatching the events.
python
import asyncio
class Event:
# Initialize the event with a name and an empty list to hold handlers
def __init__(self, name):
self.name = name
self.handlers = []
# Method to add event handlers to the event
def subscribe(self, handler):
self.handlers.append(handler)
# Asynchronously emit the event to all subscribed handlers
async def emit(self, *args, **kwargs):
tasks = [asyncio.create_task(handler(*args, **kwargs)) for handler in self.handlers]
await asyncio.gather(*tasks)
async def handle_order_received(data):
# This is a handler that processes the event data
print(f"Order received for: {data['product']}")
# Creating an event instance
order_received_event = Event('OrderReceived')
# Subscribing the handler to the event
order_received_event.subscribe(handle_order_received)
This snippet demonstrates how to emit an event, acting as the producer that triggers the event-handling process.
python
import asyncio
# Assuming the Event class and handle_order_received have been defined and set up as shown above.
async def main():
# Emit the event with the product data
await order_received_event.emit({'product': 'USB-C Cable'})
# Run the main function to start the event emission
asyncio.run(main())
# Note: If using a Jupyter notebook, replace 'asyncio.run(main())' with:
# await main()
Code language: PHP (php)
Explanatory Notes
- Event Class: Serves as a framework for creating events, subscribing handlers to these events, and emitting the events to all subscribed handlers asynchronously. This allows the system to handle multiple events and responses concurrently, improving response time and system throughput.
- Handlers: Functions or methods that are called when an event is emitted. They are designed to respond to specific types of data or events passed to them.
- Producer: The part of the system that initiates the event. In the second snippet, the main function acts as a producer by emitting an order received event.
- Consumer: The handlers that react to the event, such as handle_order_received, which processes the event data.
Data Lakes: Integrating a data lake facilitates unified data access across disparate systems. For example, a data lake using Amazon S3 can be set up to store varied data formats which can be accessed using different computing services for analysis.
When to use the data lake approach
A possible case scenario would be that of a national retail chain struggling to efficiently utilize data gathered from various store operations due to its diverse formats and siloed storage.
python
import boto3
# Initialize a session using Amazon S3
s3 = boto3.resource('s3')
# Create a new bucket
s3.create_bucket(Bucket='MyDataLake')
# Upload data to the bucket
data = open('example.txt', 'rb')
s3.Bucket('MyDataLake').put_object(Key='example.txt', Body=data)
Code language: PHP (php)
Shared Services: This involves using a single instance of a service to support multiple consumers or actors across different systems.
When to use the Shared Services Pattern
Imagine a large multinational corporation with multiple departments spread across different locations globally. By implementing the shared services pattern, they can consolidate their administrative functions like IT support, HR, finance, and procurement into centralized units. This approach enables the company to streamline processes, reduce duplication of efforts, improve efficiency, and achieve cost savings while ensuring consistent service quality across the organization.
Here’s a simple example of a REST API that serves multiple domains.
python
from flask import Flask, jsonify
app = Flask(__name__)
@app.route('/api/data')
def get_data():
# Simulate data retrieval from shared service
data = {"message": "This is shared data accessible to all domains"}
return jsonify(data)
if __name__ == '__main__':
app.run(port=5000)
Code language: PHP (php)
Anti-Corruption Layer Approach: The Anti-Corruption Layer (ACL) is another pattern that is useful in safeguarding system integrity during the integration of new technology with old, or different, systems. This strategic layer acts as a translator, adapting and converting data to prevent disruption and maintain the smooth operation of the core system.
When to use an Anti-Corruption Layer Approach
Imagine a financial institution that’s integrating a cutting-edge CRM system with an older, less agile banking transaction system. These systems don’t speak the same language in terms of data and operations. An ACL can bridge this gap by translating outdated data formats into a structure that the new CRM can understand, ensuring seamless communication and robust protection for the newer system.
Steps for Implementing an ACL
- Identify Points of Interaction: Pinpoint where the systems need to connect.
- Understand the Differences: Recognize how the data and processes vary between systems.
- Build the ACL: Create the necessary adapters or gateways to mediate data flow.
- Keep the ACL Independent: Ensure the ACL stands apart from both the core system and the external systems.
- Thoroughly Test the ACL: Verify it translates data correctly and can handle any discrepancies.
Tools:
Standardized Data Formats: Using JSON or XML ensures data is uniformly understood. For example, JSON is widely used for data interchange. It’s important to follow specific conventions, such as using camelCase notation for key names in JSON to maintain consistency. Having an international standard in the data format is key, especially in the use of ACL so as to simplify the logic and facilitate integration.
json
{
"name": "John Doe",
"email": "john.doe@example.com",
"roles": ["user", "admin"]
}
Code language: JavaScript (javascript)
API Gateways: An API Gateway serves as a critical intermediary, managing interactions between clients and services by routing requests, enforcing security protocols, and optimizing traffic and API performance. In the context of “Shared Services,” the API Gateway centralizes common functionalities used by different parts of an organization, enabling more efficient service management and scalability.
Here’s an example configuration snippet for an API Gateway (using AWS):
yaml
AWSTemplateFormatVersion: '2010-09-09'
Resources:
MyApiGateway:
Type: AWS::ApiGateway::RestApi
Properties:
Name: ExampleAPI
Code language: PHP (php)
Mapping Tools:
In the context of cross-domain interoperability, tools such as Apache NiFi and Informatica are particularly useful because they can integrate systems that are otherwise incompatible or separated by differing domains, such as security, organizational, or technological boundaries. Here are some ways in which they support cross-domain interoperability:
- Data Transformation: These tools convert data between various formats (e.g., from CSV to JSON, XML to Avro, etc.), making it easier to integrate systems that use different data standards.
- Secure Data Transfer: Encrypting data flows helps manage secure connections, ensuring that data transferred across domains is protected.
- Protocol Conversion: Both tools can interact with different network protocols (HTTP, TCP, FTP, etc.), facilitating communication between systems that utilize different networking standards.
- Workflow Automation: By automating data flows, these tools enable consistent processing and transfer of data across systems without manual intervention, reducing errors and increasing efficiency.
- System Agnosticism: They can connect to a wide range of systems and data sources, acting as a bridge in environments with diverse technological ecosystems.
Before using any commercial product or tool it’s key to analyze the complexity and uniqueness of the systems involved, in order to understand the pros and cons of the different out-of-the-box solutions.
Main Takeaways and Benefits of CDI
Adopting a Cross-Domain Interoperability (CDI) approach greatly improves the openness and accessibility of data and resources. This method allows different systems to interact seamlessly, fostering environments that are inclusive and open. Such ecosystems provide broad access to data and resources, encouraging a diverse group of stakeholders to leverage this information for innovation. This enhances knowledge sharing and leads to the creation of solutions that truly meet the needs of end-users. Ultimately, CDI supports the evolution of industries and sectors by ensuring they can meet modern challenges effectively.
Here is a breakdown of the main benefits to consider:
- Tackling IT Complexity: CDI streamlines how different systems interact, cutting down on the need for complex custom solutions.
- Boosting Efficiency and Integration: By enabling smooth data exchanges and automating processes, CDI helps operations run more smoothly and reduces the likelihood of mistakes.
- Creating Future-Ready Apps: The adaptability and flexibility of CDI allow organizations to easily embrace new technologies.
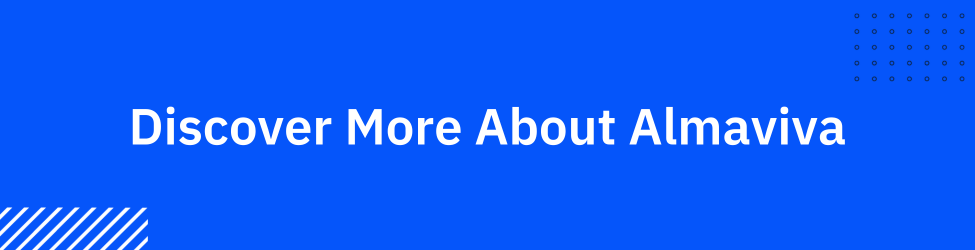