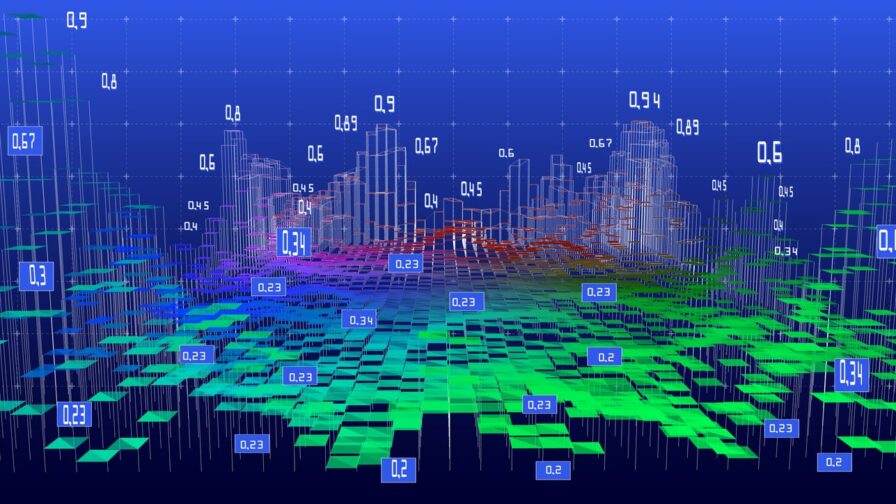
What Are Python Environment Variables?
Python environment variables are dynamic named values stored in the system that software programs use to change behavior without altering the code. They are primarily used to manage the settings of various applications, libraries, and modules in Python.
Environment variables are a type of variable that is set outside the program, in the operating system, and passed to the program when it starts. They can be accessed by the os module in Python using the os.environ object, which is a dictionary-like object that allows you to retrieve the value of the environment variable by its name.
Python environment variables have many uses. They can hold global settings, system paths, API keys, database credentials, and other sensitive information. For instance, you might want to have different settings for development and production environments. Instead of hardcoding these settings into your Python code, you could set them as environment variables.
Importance of Environment Variables in Python
Environment variables play a critical role in the development and production of Python applications. They provide a way to configure your Python application based on the environment you’re running it in. This helps to keep your application flexible and easy to configure without having to change the source code.
Python environment variables are useful for handling sensitive data such as passwords, API keys, and database URIs. By storing these sensitive pieces of information in environment variables, you can keep them out of your codebase. This can reduce the chance of leaking sensitive information if your code is made public or falls into the wrong hands. However, you should note that environment variables are also not completely secure, and sensitive data should be encrypted or securely stored by secret management systems.
Environment variables can also help you manage dependencies in your Python application. They can be used to specify the locations of libraries or other resources that your application needs to run. This can be useful when you’re developing on multiple machines or when you’re working with a team.
Recommended article: automate everything with Python
Common Pitfalls and How to Avoid Them
Let’s look at some of the most common mistakes made by developers when working with Python environment variables.
Hardcoding Sensitive Information
A major mistake is hardcoding sensitive information directly into the application code. This might seem like a convenient solution at first, but it’s a major security risk. If your application’s source code is ever exposed—whether through a data breach or because it’s open source—any sensitive data hardcoded into it will be laid bare for anyone to see. This can lead to serious consequences, from data theft to malicious attacks on your systems
Hardcoding sensitive data also makes your application less flexible. If you ever need to change that data—for instance, switching to a different database or changing your API keys—you’ll have to modify and redeploy your application code. This is both time-consuming and error-prone, especially in large applications.
To avoid this pitfall, always store sensitive data in environment variables, and access them through your code. Python’s os.environ object makes this easy. You can set environment variables in your operating system or application runtime, and your Python code can access them like so: os.environ[‘MY_VARIABLE’]. This way, your sensitive data stays secure and your code stays flexible.
Inconsistent Naming Conventions
Consistent naming conventions are important for avoiding confusion and bugs. In Python, environment variables are case-sensitive. This means that os.environ[‘MY_VARIABLE’] and os.environ[‘my_variable’] are two entirely different variables. If you’re not consistent with your capitalization, you can easily end up with two variables where you intended to have one, or vice versa.
In addition, it’s important to use clear, descriptive names for your variables. Names like DB or KEY might make sense to you when you’re writing the code, but they can be confusing for others (or even for you, six months down the line). Instead, use names that clearly indicate what the variable is used for, like DATABASE_URL or API_KEY.
Consider using a naming convention that separates words with underscores (_), like MY_VARIABLE_NAME. This makes your variable names easier to read and avoids confusion with Python’s syntax.
Recommended article: Python vs Julia for Data Science
Not Handling Missing Variables Gracefully
When a necessary variable isn’t set, many applications will crash with only a KeyError. This is a poor user experience, and it’s also a missed opportunity to guide the user towards a solution.
Instead of letting your application crash, you should handle missing environment variables gracefully. This means checking whether a variable is set before you try to use it, and providing a helpful error message if it’s not. Python’s os.getenv() function is suitable for this: it allows you to access an environment variable and specify a default value to use if the variable isn’t set.
Here’s an example of how you might use os.getenv() to handle a missing database URL:
database_url = os.getenv(‘DATABASE_URL’)
if database_url is None:
raise RuntimeError(‘The DATABASE_URL environment variable is not set. Please set it and try again.’)
In this example, if DATABASE_URL isn’t set, the application will raise an error with a clear, helpful message. This way, the user knows exactly what the problem is and how to fix it.
Overlooking Environment Variable Scope
Environment variables are global to the process they’re set in, which means they can be accessed from anywhere in your code. This makes them versatile, but it also means that if you’re not careful, you can end up with multiple parts of your codebase relying on the same variable, leading to tight coupling and making your code harder to understand and maintain.
To avoid this, be mindful of where and how you use environment variables. Try to limit their use to configuration code and avoid using them in business logic. If multiple parts of your code need the same piece of data, consider passing it as a parameter or storing it in a shared object, rather than relying on an environment variable.
Environment variables are shared between your application and any subprocesses it spawns. This means that if you change an environment variable in your application, it can affect your subprocesses as well. To avoid unexpected side effects, always use the os.environ.copy() function to create a copy of the environment for your subprocesses.
Conclusion
While Python environment variables are a powerful tool, they come with several potential challenges. By understanding and avoiding these pitfalls, you can use environment variables effectively and securely, making your Python applications more robust, flexible, and user-friendly.